In this tutorial, we’ll discuss Arduino Timers from the very basic concepts all the way to implementing Arduino timer-based systems. We’ll start off by discussing what is a timer, how they work, and what are different timer operating modes. You’ll learn all Arduino timer mechanics and how to properly set up timer-based systems.
We’ll create a couple of Arduino Timer Example Code Projects in this tutorial to practice what we’ll learn all the way through. And finally, we’ll draw some conclusions and discuss some advanced tips & tricks for Arduino timers that will definitely help you take some guided design decisions in your next projects. Without further ado, let’s get right into it!
Table of Contents
- Arduino Timers
- Arduino Timers – Timer Mode
- Arduino Timers – Counter Mode
- Arduino Timers – PWM (Output Compare)
- Arduino Timers – Input Capture Unit
- Arduino Timers Libraries
- Wrap Up
Arduino Timers
Timer modules in Arduino provide precise timing functionality. They allow us to perform various tasks, such as generating accurate delays, creating periodic events, measuring time intervals, and meeting the time requirements of the target application.
Each Arduino board has its target microcontroller that has its own set of hardware timers. Therefore, we always need to refer to the respective datasheet of the target microcontroller to know more about its hardware capabilities and how to make the best use of it.
1. Arduino Hardware Timers
Arduino UNO (Atemga328p) has 3 hardware timers which are:
- Timer0: 8-Bit timer
- Timer1: 16-Bit timer
- Timer2: 8-Bit timer
Those timer modules are used to generate PWM output signals and provide timing & delay functionalities to the Arduino core, and we can also use them to run in any mode to achieve the desired functionality as we’ll see later on in this tutorial.
Each hardware timer has a digital counter register at its core that counts up based on an input clock signal. If the clock signal is coming from a fixed-frequency internal source, then it’s said to be working in timer mode. But if the clock input is externally fed from an IO or any async source, it’s said to be working as a counter that counts incoming pulses.
2. Why Do We Use Timers?
It’s way more efficient to use hardware timers instead of software solutions, especially replacing things like delay() function, periodic event handling, PWM signal generation, and many other applications. Hardware timer modules can operate in one of many modes, each of which has its own applications and use cases as we’ll discuss hereafter in the next section.
Those are the most common use cases for hardware timers:
- Generating time interval delay between events
- Periodic events handling
- Measuring the time between events
- Counting external or internal events/pulses
- Generating PWM output signals
By configuring a hardware timer, we can get it to achieve any of the applications mentioned above as we’ll see when we discuss the timer operating modes.
3. Arduino Timer Prescaler
A prescaler in a hardware timer module is a digital circuit that is used to divide the clock signal’s frequency by a configurable number to bring down the timer clock rate so it takes longer to reach the overflow (maximum count number).
This is really useful to control the maximum time interval that can be generated using the timer module, the PWM output frequency, or the range of time that can be measured using the timer module.
Running the timer module at the system frequency is good for resolution but will generate so many timer overflow interrupts that needs extra care in your code. Hence, using a prescaler can be useful to avoid this situation in the first place if needed.
In timer mode, the timer module will have the internal clock of the system as a clock source and it passes through the prescaler as shown below. You can programmatically control the division ratio of the prescaler to control the timer input clock frequency as you need.

The timer prescaler divider values differ from one timer module to another and it’s clearly stated in the datasheet for each timer module (Timer0, 1, and 2).
4. Arduino Timer Interrupts
Arduino timers provide different interrupt signals for various events. Such as timer overflow, when a timer reaches its maximum count value (255 for 8-Bit, and 65535 for 16-Bit timers). It fires an overflow interrupt, rolls back to zero, and starts counting up again.
There is also two output compare registers in each timer (COMPA and COMPB). When the timer register’s counter value reaches COMPA value, it drives the OCnA pin HIGH or LOW depending on your configurations, and it also fires a COMPA interrupt (if enabled). And the same goes for COMPB.
This is an interactive simulation of how timer interrupt events are generated (Timer Overflow, COMPA, and COMPB).
Each timer interrupt signal can be enabled or disabled individually and has its own interrupt vector address.
5. Arduino Timers Comparison
This is a summarized table for Arduino UNO (Atmega328p) timers, differences between them, capabilities, operating modes, interrupts, and use cases.
Timer0 | Timer1 | Timer2 | |
Resolution | 8 Bits | 16 Bits | 8 Bits |
Used For PWM Output Pins# | 5, 6 | 9, 10 | 11, 3 |
Used For Arduino Functions |
delay() millis() micros() | Servo Functions | tone() |
Timer Mode | ✓ | ✓ | ✓ |
Counter Mode | ✓ | ✓ | ✓ |
Output Compare (PWM) Mode | ✓ | ✓ | ✓ |
Input Capture Unit Mode | – | ✓ | – |
Interrupts Vectors |
TIMER0_OVF_vect TIMER0_COMPA_vect TIMER0_COMPB_vect |
TIMER1_OVF_vect TIMER2_COMPA_vect TIMER1_COMPB_vect TIMER1_CAPT_vect |
TIMER2_OVF_vect TIMER2_COMPA_vect TIMER2_COMPB_vect |
Prescaler Options | 1:1, 1:8, 1:64, 1:256, 1:1024 | 1:1, 1:8, 1:64, 1:256, 1:1024 | 1:1, 1:8, 1:32, 1:64, 1:128, 1:256, 1:1024 |
Playing with any timer configurations can and will disrupt the PWM output channels associated with that module. Moreover, changing the configurations of timer0 will disrupt the Arduino’s built-in timing functions ( delay, millis, and micros). So keep this in mind to make better design decisions in case you’ll be using a hardware timer module for your project. Timer1 can be the best candidate so to speak.
Arduino Timers – Timer Mode
The timer module in timer mode is configured to have the internal system clock as the clock source with multiple prescaler options. It’s generally used to generate fixed time interval interrupts to insert time delays between events or to execute periodic events at fixed time intervals.
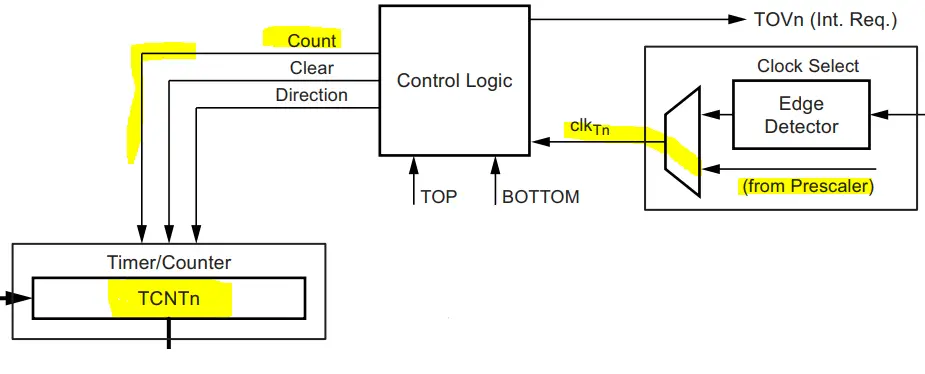
In time mode, the timer module will keep counting (0 to 255 or 65535 depending on resolution). At overflow, a timer overflow interrupt is fired and the timer rolls over back to zero and starts counting again.
Arduino Timer Equations
Timer modules in microcontrollers have a general equation which is as follows:

The desired output time interval (TOUT) is equal to the number of timer ticks multiplied by the single tick time. The timer’s tick time is determined by the input clock frequency and the prescaler ratio that you’ve selected. Therefore, the general timer equation can be expressed like this:

And that’s the equation we’ll be using to design timer-based applications (e.g. time interval delays, periodic tasks execution, and much more). You should note that the maximum TOUT is defined by the prescaler ratio configuration. Changing the prescaler will allow us to raise the maximum allowable TOUT time interval generation with the timer module before it overflows.
For example, Timer1 in Arduino UNO is clocked at 16MHz. With a prescaler of 1:1, the Maximum TOUT can be achieved by setting the TicksCount to its maximum value of 65536. This will give us TOUT(Max) = (1×65536)/16M = 4.01ms.
If you need to generate a larger TOUT time interval with that timer module @ 16MHz clock, you need to choose another option for the prescaler. Let’s say you’d like to generate a 500ms timer interrupt as a time-base for your system. This means, the TOUT(MAX) needs to be >500ms.
Therefore, setting the prescaler ratio to 1:256 will be the best option. Because it’ll allow a maximum TOUT(MAX) of = (256×65536)/16M = 1.049s. Which is more than the 500ms requirement so it becomes achievable by selecting this larger prescaler ratio. Let’s now discuss how to use the general timer equation and do the required calculations to be able to configure the timer module properly.
Arduino Timer Calculations
The best way to demonstrate timer module calculations is to consider a practical example use case and walk through the calculation process step by step. And that’s what we’re going to do in this section.
Let’s say you’d like to generate a periodic timer interrupt every 100ms and use it as a time base for your system. We’ll be using the Timer1 module which is clocked at 16MHz (in Arduino UNO boards).
Step 1) Select a suitable prescaler divider. The required TOUT is 100ms. The TOUT(MAX) should be > 100ms. Therefore, selecting a prescaler of 1:64 will be sufficient. Because at 1:64 prescaler, the TOUT(MAX) = (64×65536)/16M = 262ms. Which is definitely above the required 100ms time interval.
Step 2) Using the general timer equation, plug in the (TOUT value, Prescaler divider, and CLK frequency). Then solve the equation for the TicksCount value.

Step 3) TicksCount = 25,000 ticks. And that’s the output of the calculation that we’ll use thereafter to program the timer module to generate the desired 100ms timer interrupt event.
Arduino Timer Programming
After calculating the required timer TicksCount to achieve the desired TOUT time interval for timer interrupt events, we can go about programming the Arduino timer module in two different ways.
1- Timer Preloading
First method is to preload the timer register (TCNTx) with a value in such a way it reached overflow (65535) after only TicksCount ticks. For the previous 100ms example, the TicksCount turned out to be 25000 ticks. Therefore, the timer preload value = 65535-25000 = 40535.
By writing 40535 to the TCNTx register, we guarantee that it’s going to tick 25000 ticks to reach the overflow state. In the timer overflow interrupt ISR handler, we’ll also need to preload the TCNTx register with the same value and keep repeating over and over again.
Here is an Arduino code example for Timer Preloading.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/* * LAB Name: Arduino Timer Preloading * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ ISR(TIMER1_OVF_vect) { TCNT1 = 40535; // Timer Preloading // Handle The 100ms Timer Interrupt //... } void setup() { TCCR1A = 0; // Init Timer1 TCCR1B = 0; // Init Timer1 TCCR1B |= B00000011; // Prescalar = 64 TCNT1 = 40535; // Timer Preloading TIMSK1 |= B00000001; // Enable Timer Overflow Interrupt } void loop() { // Do Nothing } |
2- Timer Compare Registers
An easier way to achieve the same goal without disrupting the timer’s TCNTx register’s value would be to use the output compare match interrupt events. This is probably the best way to implement timer-based interrupt events. Because it gives you two (COMPA and COMPB) registers to generate two independent time interrupt events using the same timer module.
Considering the previous 100ms time interval interrupt example, we need the timer to tick 25000 ticks to get the 100ms periodic interrupt. So, we’ll use COMPA compare register, enable its interrupt, and set its value to 25000. When a compare match occurs (when TCNT1 = OCR1A), an interrupt is fired. And that’s the 100ms periodic interrupt we want.
To keep it running at the same 100ms rate, we need to update the COMPA value because the timer’s count has now reached 25000. Therefore, we need to add 25000 ticks to the COMPA value. Don’t worry about overflow, at 65535, the register will roll over back to zero exactly like the timer’s TCNTx register does. So it’s going to work flawlessly all the time.
And that’s it! Here is an Arduino code example for Timer Compare Match Interrupt.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
/* * LAB Name: Arduino Timer Compare Match Interrupt * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ ISR(TIMER1_COMPA_vect) { OCR1A += 25000; // Advance The COMPA Register // Handle The 100ms Timer Interrupt //... } void setup() { TCCR1A = 0; // Init Timer1 TCCR1B = 0; // Init Timer1 TCCR1B |= B00000011; // Prescalar = 64 OCR1A = 25000; // Timer CompareA Register TIMSK1 |= B00000010; // Enable Timer COMPA Interrupt } void loop() { // Do Nothing } |
Arduino Timer Calculator & Code Generator Tool
To make things much easier & quicker for you, here is an Arduino Timer Interrupt Calculator & Code Generator Tool. This tool will take your desired time interval, the timer module’s number, and the type of interrupt signal you’d like to use. And it’ll give you a complete Arduino startup sketch ready for your project. You can either copy & paste it as is or just get the configurations, timer prescaler, and timer preload value.
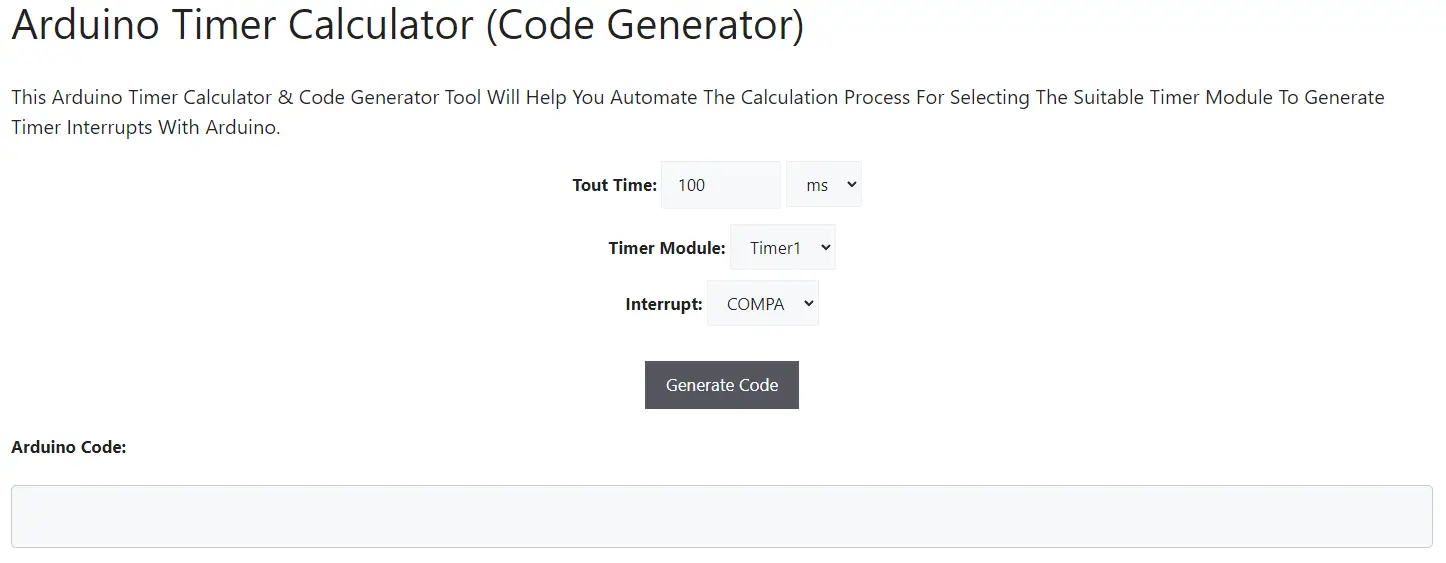
Both methods shown in this section (Timer Preloading & Timer Compare Match), more in-depth demonstration, more code examples & testing, and how to use the Arduino timer calculator & code generator, all can be found in the dedicated tutorial linked below. This is the ultimate guide for Arduino Timer Interrupts that you definitely need to check out.
This tutorial will give you more in-depth information about Arduino Timer interrupts and how to set up timer interrupts for periodic events. You’ll also learn how to use our timer code generator for different interrupt types (COMPA, COMPB, and OVF) with multiple code example projects.
Arduino Timers – Counter Mode
The timer module in counter mode is configured to have an external pin as the clock source with multiple prescaler options. It’s generally used to count external input pulses or measure an input signal by counting its rising or falling edges over a fixed time interval.
There are so many applications and use cases for timer modules in counter mode as we’ll see later on. Here is the block diagram for the hardware timer in counter mode.
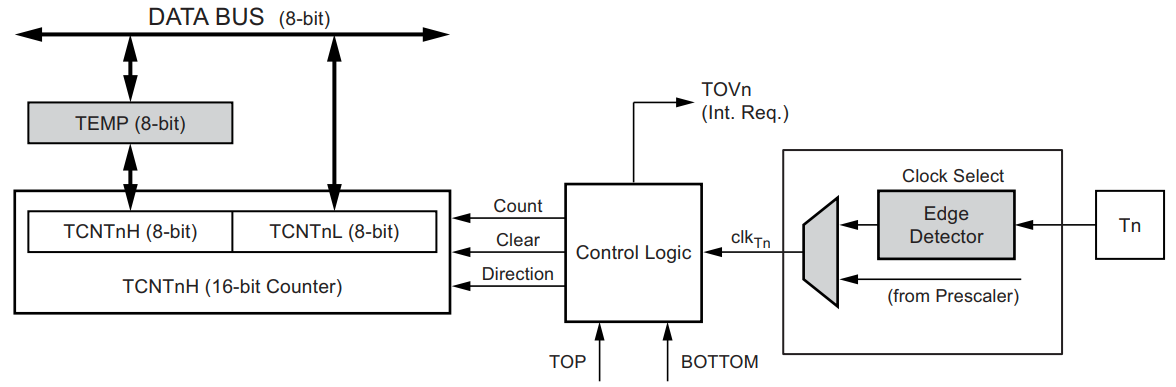
As you can see, the Tn pin is the input pin to the timer’s clock source. This is the major difference between a hardware timer working in Counter Mode vs Timer Mode. The Timer input pin (Tn) can be hooked up to a push button and we can use it to count the pulses generated when we click on the button.
The timer module in counter mode can be set to count up each RISING or FALLING edge on the Tn input pin.
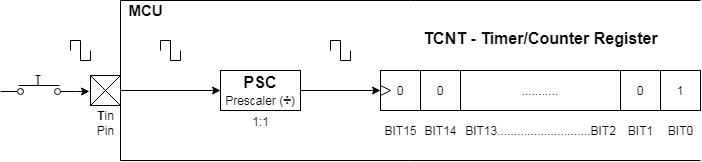
You can refer to the Arduin UNO Pinout Guide to identify all timer input pins (Tn). Where T0 is the Timer0 input pin and T1 is the Timer1 input pin. In Arduino UNO (Atmega328p), the T0 & T1 pins are Arduino IO pins (4 & 5) respectively. Also note that: the Counter0 register (TCNT0) is 8 bits, while the Counter1 register (TCNT1) is 16 bits.
Timer2 doesn’t have an input pin so it doesn’t operate in counter mode.
Arduino Counter Timer Mode Programming
To set an Arduino Timer module to operate in counter mode, we’ll use the clock selection bits in the TCCRxB register. Here are the counter mode clock options for the least significant 3 bits in the TCCRxB register (as stated in the datasheet).
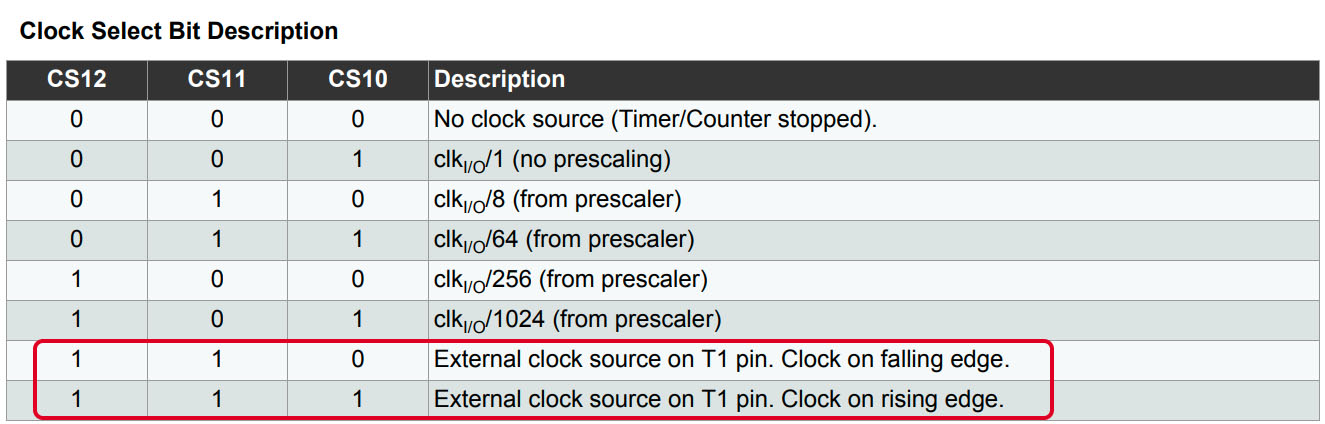
Here is an example of an Arduino Counter Timer Code that sets Timer1 to operate in Counter Mode (counts up every RISING edge on the T1 pin). The counter register’s value is sent to the PC over the serial port 4 times per second.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
/* * LAB Name: Arduino Timer (Counter Mode) * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ void setup() { TCCR1A = 0; // Init Timer1A TCCR1B = 0; // Init Timer1B TCCR1B |= B00000111; // External Clock on T1 Pin (RISING) TCNT1 = 0; Serial.begin(9600); } void loop() { Serial.print("Counter Ticks = "); Serial.println(TCNT1); delay(250); } |
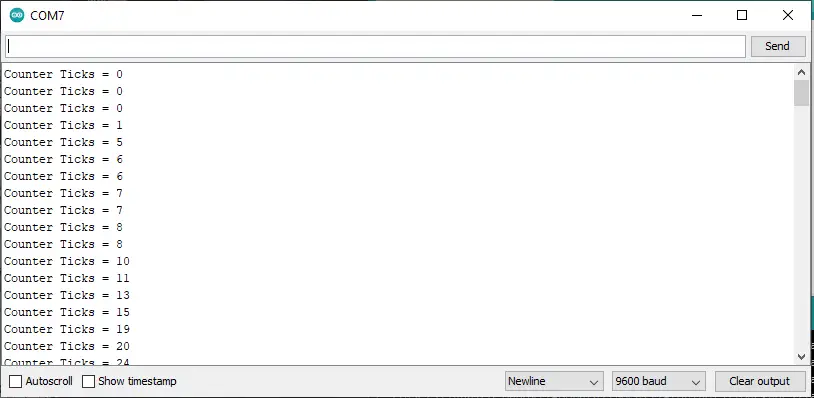
For more examples and demonstrations of Arduino Timers (Counter Mode), it’s highly recommended to check out its dedicated tutorial linked below. It’ll give you more information, code examples, and applications for Arduino Timers in Counter Mode.
This tutorial will give you more in-depth information about Arduino Timers ( in Counter Mode). How to configure Arduino Timers to operate in Counter mode, and what are the use cases & applications for Counter Mode? As well as a couple of practical Arduino code example projects.
Note that Arduino Timers in Counter Mode depend on an external clock source which is the Tn input pin. This IO pin is obviously susceptible to all sorts of noise (like the Button Bouncing if you’re using a push button hooked to the Tn pin). Therefore, it’s your responsibility to clean up the signal on a hardware level. There is no way to debounce or filter the signal in the software.
And state change on the Tn pin will be considered as a clock cycle that will cause the counter register to count up even if it was just a glitch or noise. Software algorithms can’t fix this issue. However, a simple RC Filter on the Tn input pin will prevent this issue in the first place.
Arduino Timers – PWM (Output Compare)
The timer module in output compare mode (PWM) is configured to have an internal clock source with multiple prescaler options. It’s generally used to generate PWM output signals on the OCnx pins (OCnA & OCnB), where n is the timer module’s number.
Here is a screenshot for the block diagram of the output compare unit hardware within timer modules in Arduino (Atmega328p) microcontroller.
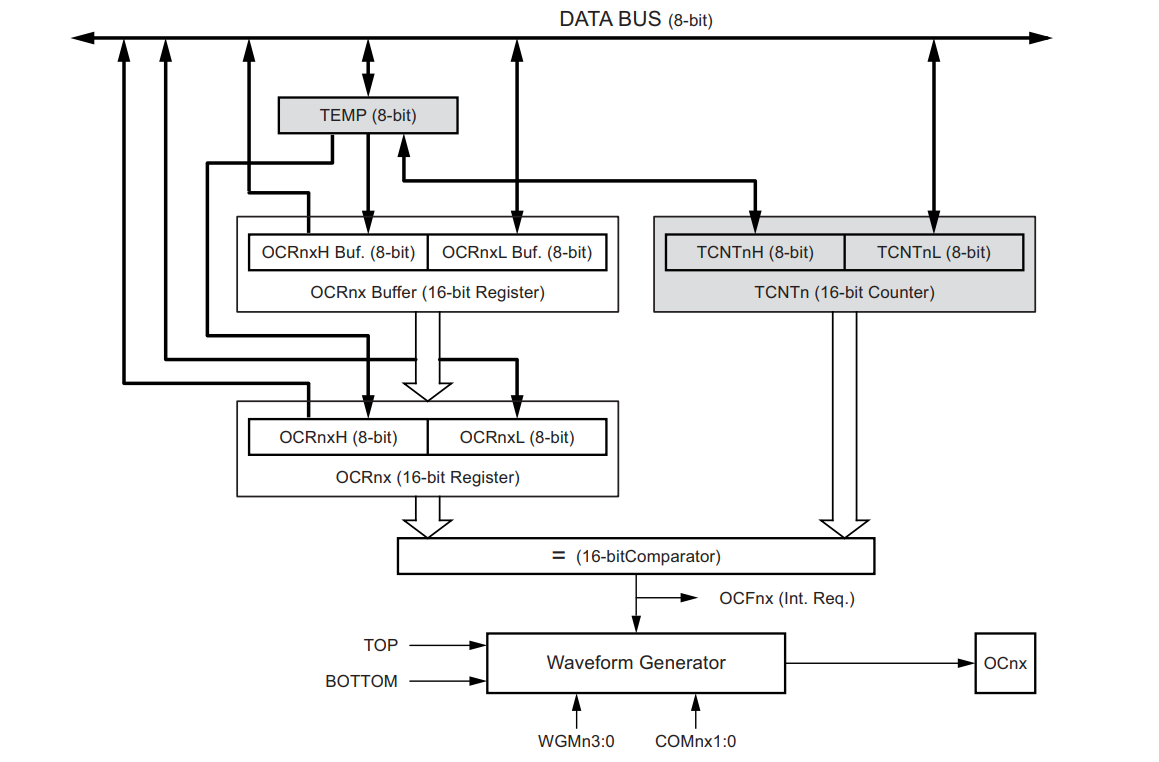
The timer module will be free running all the time, and its value (TCNTn) is continuously compared against the OCRnx register (which is double-buffered to eliminate PWM glitches). When the TCNTn matched the OCRnx register’s value, a compare match event is triggered which drives the OCnx output pin HIGH or LOW depending on your configuration.
At the overflow of the TCNTn counter register, the OCnx pin state is flipped. This gives us a PWM output signal on the OCnx pin whose duty cycle can be easily controlled by the OCRnx register. For each timer, there are two PWM output pins as stated earlier (OCnA & OCnB).
Arduino Output Compare (PWM Mode) Programming
There is a built-in Arduino function for PWM output control which is the analogWrite() function. That makes it much easier to generate PWM output signals with Arduino. Which is very useful in countless applications.
You can learn more in-depth information about Arduino PWM signal generation & create a couple of practice example projects by following the dedicated tutorial linked below. It’s the ultimate guide for Arduino PWM that you need to keep for your reference.
This tutorial will provide you with more in-depth information about Arduino PWM output. Starting from the basics of PWM signal, its frequency, duty cycle, and resolution, and will discuss in detail how it works and how to use it in various Arduino control projects.
Arduino Timers – Input Capture Unit
The timer module in input capture mode is configured to have an internal clock source with multiple prescaler options. It’s generally used to capture the timer counter value at certain events on the input capture pin (RISING or FALLING edges). The (Input Capture Unit) is also referred to as ICU for short.
The timer module will be free running all the time, and its value (TCNTx) is captured and saved into the input capture register (ICRx) immediately when the input capture pin state has changed. This is like taking a time stamp from the running timer at certain pin state change events (on the ICPn pin).
Input Capture Unit (ICU) Hardware
There are so many applications and use cases for timer modules in input capture mode as we’ll see later on. Here is the block diagram for the hardware timer input capture unit.
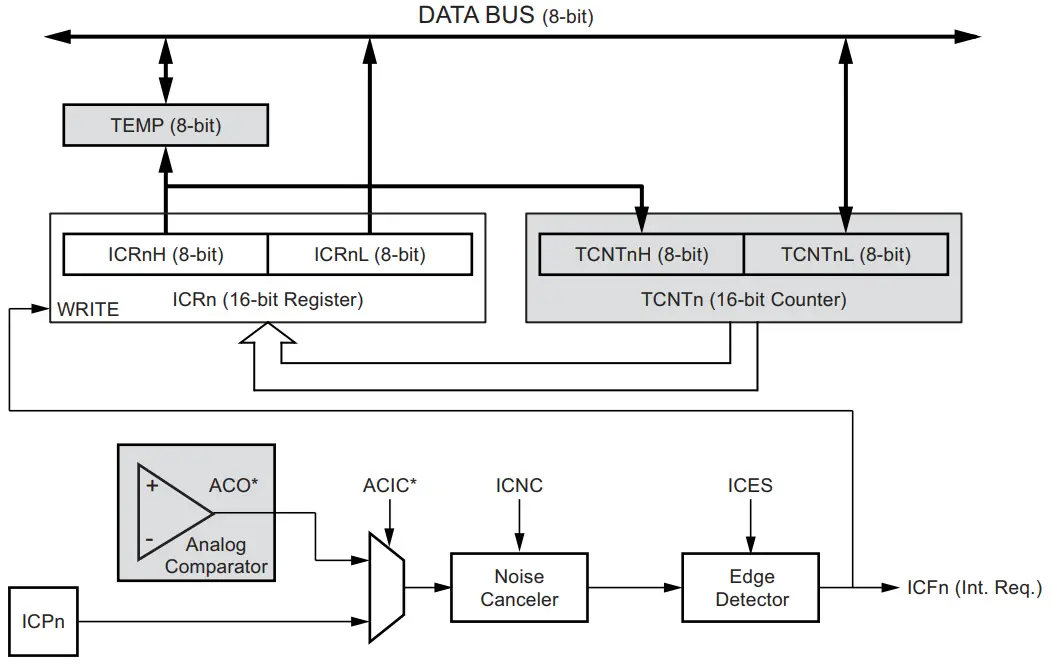
As you can see in the ICU hardware diagram, the timer counter register (TCNTx) is copied to the input capture register (ICRx) when an input capture edge is detected. It can either be coming from the ICPx external pin or from the internal analog comparator output (ACO).
The ICPx pin can trigger an input capture event on RISING or FALLING edges. When an input capture event is triggered, the current timer/counter register (TCNTx) value is copied to the input capture register (ICRx), and an interrupt is fired, if enabled. The Arduino input capture interrupt vector is TIMER1_CAPT_vect.
Input Capture Unit Frequency Measurement
As stated earlier, the ICU mode is typically used to get time stamps of a free running timer module at certain events on an external pin (ICPx). Which can be very useful for applications like frequency measurement. We can set up the ICU to trigger a capture event on every RISING edge on the ICPx pin to measure an external signal’s frequency.
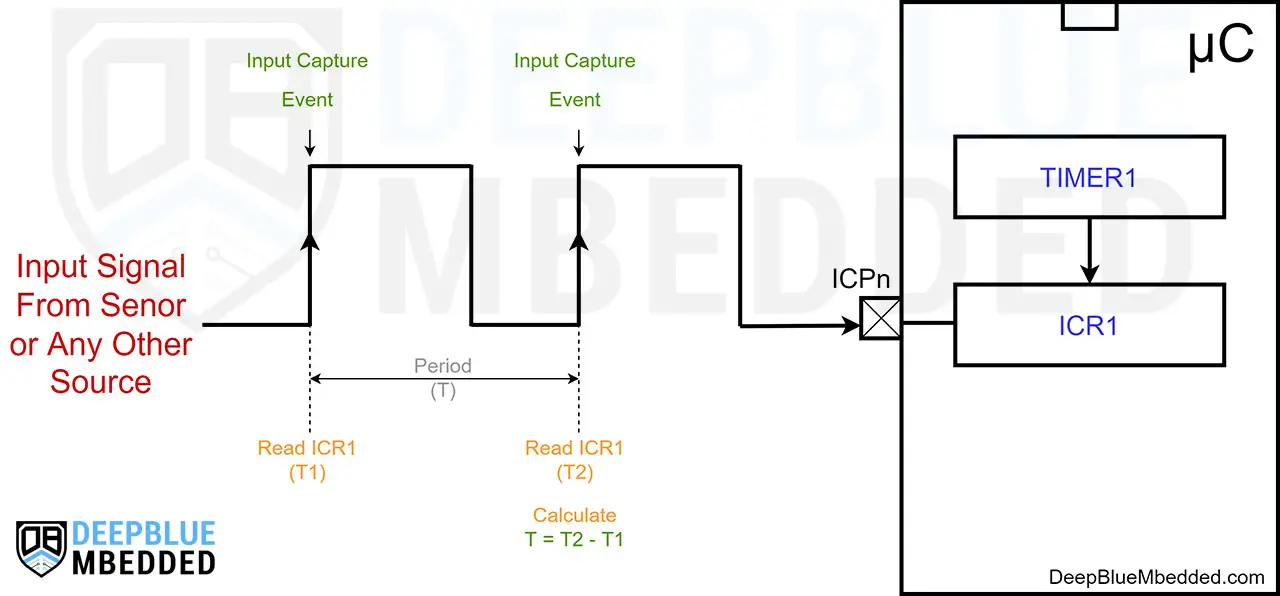
At the first edge, we’ll save the ICRx register reading into a variable (T1). At the second edge, we’ll save ICRx into (T2). And to get the signal’s period, we just subtract the time stamps as follows: ( T = T2 - T1). Just pay attention to timer overflow events because if T2 is smaller than T1, the resulting period (T) will be negative which is not correct. We need to add ( Number of TimerOVF * 65536) to T2 to get the correct absolute timestamp difference.
Duty Cycle (Pulse Width) Measurement With ICU
Measurement of an external signal’s duty cycle (pulse width) requires that the trigger edge is changed after each capture event. Changing the edge sensing must be done as early as possible after the ICRx register has been read. After a change of the edge, the input capture flag (ICFx) must be cleared by software (writing a logical one to the bit location).
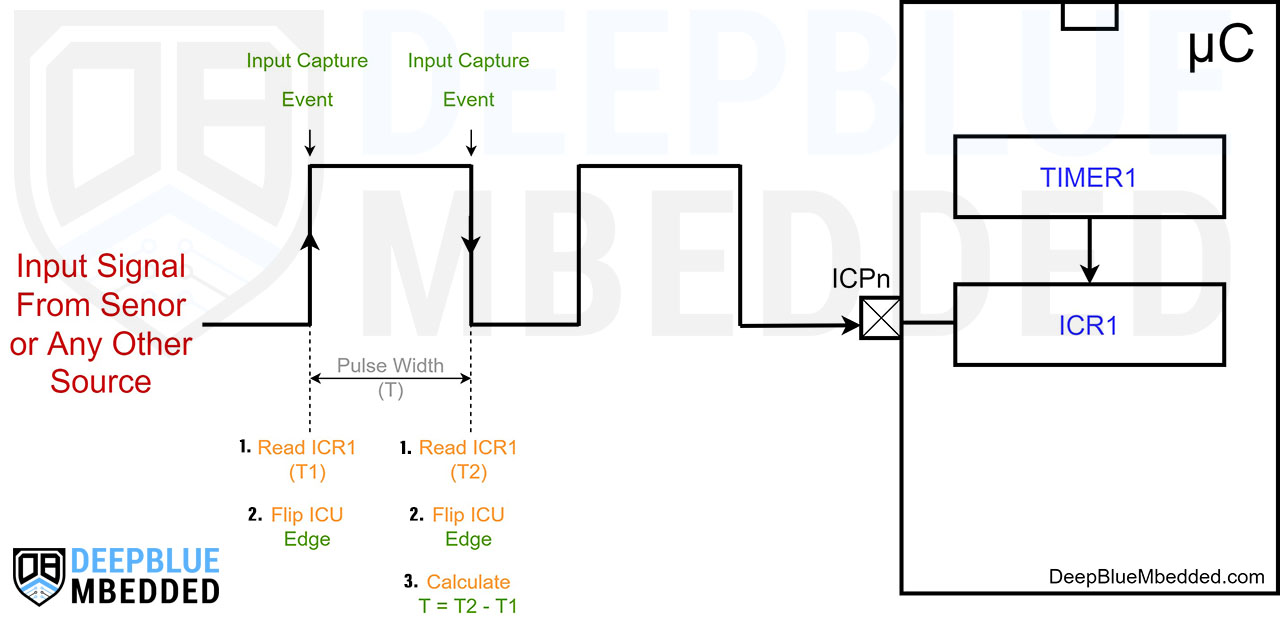
You can refer to the Arduin UNO Pinout Guide to identify all timer input capture pins (ICPn).
In Arduino UNO (Atmega328p) microcontroller, Only Timer1 has an input capture unit (timer mode). Which has an input capture pin (ICP1) that corresponds to Arduino UNO IO pin8.
To learn more about Arduino Input Capture Unit and create a couple of practice projects, you need to check out the tutorial linked below. This is a very important topic in this Arduino Programming Tutorials Series and will be used in a lot of sensor interfacing tutorials & projects. So make sure to get the hang of it.
This tutorial will give you more in-depth information about Arduino Timers (in input capture Mode). How to configure Arduino Timers to operate in ICU mode, and what are the use cases & applications for ICU? As well as a couple of practical Arduino code example projects.
Arduino Timers Libraries
There is a handful of community-contributed Arduino Timer libraries that eases the process of configuring the Arduino internal hardware timers and provide some useful APIs (functions) to do a lot of things. However, I’ll only discuss the most common two libraries that will satisfy most of your needs in most projects.
You need both of them actually, the decision of which to use in which project depends on your needs. The arduino-timer.h library excels in handling timing functions, concurrent tasks, and such. While the Arduino TimerOne.h library excels at giving you the flexibility to control the PWM outputs of Timer1 (only pins 9 & 10). You can use it to generate 20kHz PWM outputs at a very good resolution,
1- Arduino-Timer Library
The Arduino-Timer library is a community-contributed library that enables users to configure timer-based events (tasks) without the need to do register-level programming for the timer modules. It uses the built-in timer-based millis() and micros() functions, so it’s like a wrapper layer of useful APIs on top of the built-in timer-based functions.
You can follow the tutorial below for installation instructions and in-depth information & illustration for the library functions with a couple of project examples to test the library functionalities in practice.
This tutorial will give you more in-depth information about arduino-timer.h library. How to install and use Arduino-Timer library. And will also create a couple of test projects to create & handle periodic tasks with arduino-timer library (in ms & μs resolutions).
2- Arduino TimerOne Library
The Arduino TimerOne library is a community-contributed library that enables users to configure and use the 16-Bit Timer1 for generating & handling periodic interrupts and also to generate PWM signals with controllable frequency and duty cycle.
You can follow the tutorial below for installation instructions and in-depth information & illustration for the library functions with a couple of project examples to test the library functionalities in practice.
This tutorial will give you more in-depth information about Arduino TimerOne.h Library. How to install and use Arduino TimerOne library. And will also create a couple of test projects to create & handle periodic timer interrupt events, and also generate 20kHz PWM output signals with Arduino.
Parts List
Here is the full components list for all parts that you’d need in order to perform the practical LABs mentioned here in this article and for the whole Arduino Programming series of tutorials found here on DeepBlueMbedded. Please, note that those are affiliate links and we’ll receive a small commission on your purchase at no additional cost to you, and it’d definitely support our work.
Wrap Up
To conclude this tutorial, we’d like to highlight the fact that the Arduino Timers are very useful in so many applications (like timer interval interrupts generation, periodic tasks handling, measurement of signals, and more). Each timer mode has its unique set of features, working mechanics, and potential applications.
This tutorial is a fundamental part of our Arduino Series of Tutorials because we’ll build on top of it to interface various sensors and modules with Arduino in other tutorials & projects. So make sure you get the hang of it and refer to all the linked tutorials below to learn more about Arduino Timer Modes.
- Arduino Timer Mode Tutorial
- Arduino Counter Mode Tutorial
- Arduino Output Compare (PWM) Tutorial
- Arduino Input Capture Unit (ICU) Tutorial
If you’re just getting started with Arduino, you need to check out the Arduino Getting Started [Ultimate Guide] here.
This is the ultimate guide for getting started with Arduino for beginners. It’ll help you learn the Arduino fundamentals for Hardware & Software and understand the basics required to accelerate your learning journey with Arduino Programming.