In this tutorial, we’ll discuss Arduino TimerOne Library how it works, and how to use it to handle periodic tasks (functions). The Arduino TimerOne library can be easily installed within Arduino IDE itself. It provides users with useful APIs to configure and use the 16-Bit Timer1 for generating & handling periodic interrupts and also to generate PWM signals with controllable frequency and duty cycle.
We’ll create a couple of Arduino TimerOne Example Projects in this tutorial to practice what we’ll learn all the way through. Without further ado, let’s get right into it!
Table of Contents
- Arduino Timers
- Arduino TimerOne Library
- Arduino TimerOne Library Installation
- Arduino TimerOne Timer Interrupt Example
- Arduino TimerOne 20kHz PWM Example
- Arduino TimerOne Wrap Up
Arduino Timers
Timer modules in Arduino provide precise timing functionality. They allow us to perform various tasks, such as generating accurate delays, creating periodic events, measuring time intervals, and meeting the time requirements of the target application.
Each Arduino board has its target microcontroller that has its own set of hardware timers. Therefore, we always need to refer to the respective datasheet of the target microcontroller to know more about its hardware capabilities and how to make the best use of it.
1. Arduino Hardware Timers
Arduino UNO (Atemga328p) has 3 hardware timers which are:
- Timer0: 8-Bit timer
- Timer1: 16-Bit timer
- Timer2: 8-Bit timer
Those timer modules are used to generate PWM output signals and provide timing & delay functionalities to the Arduino core, and we can also use them to run in any mode to achieve the desired functionality as we’ll see later on in this tutorial.
Each hardware timer has a digital counter register at its core that counts up based on an input clock signal. If the clock signal is coming from a fixed-frequency internal source, then it’s said to be working in timer mode. But if the clock input is externally fed from an IO or any async source, it’s said to be working as a counter that counts incoming pulses.
2. Arduino Timers Comparison
This is a summarized table for Arduino UNO (Atmega328p) timers, differences between them, capabilities, operating modes, interrupts, and use cases.
Timer0 | Timer1 | Timer2 | |
Resolution | 8 Bits | 16 Bits | 8 Bits |
Used For PWM Output Pins# | 5, 6 | 9, 10 | 11, 3 |
Used For Arduino Functions |
delay() millis() micros() | Servo Functions | tone() |
Timer Mode | ✓ | ✓ | ✓ |
Counter Mode | ✓ | ✓ | ✓ |
Output Compare (PWM) Mode | ✓ | ✓ | ✓ |
Input Capture Unit Mode | – | ✓ | – |
Interrupts Vectors |
TIMER0_OVF_vect TIMER0_COMPA_vect TIMER0_COMPB_vect |
TIMER1_OVF_vect TIMER2_COMPA_vect TIMER1_COMPB_vect TIMER1_CAPT_vect |
TIMER2_OVF_vect TIMER2_COMPA_vect TIMER2_COMPB_vect |
Prescaler Options | 1:1, 1:8, 1:64, 1:256, 1:1024 | 1:1, 1:8, 1:64, 1:256, 1:1024 | 1:1, 1:8, 1:32, 1:64, 1:128, 1:256, 1:1024 |
Playing with any timer configurations can and will disrupt the PWM output channels associated with that module. Moreover, changing the configurations of timer0 will disrupt the Arduino’s built-in timing functions ( delay, millis, and micros). So keep this in mind to make better design decisions in case you’ll be using a hardware timer module for your project. Timer1 can be the best candidate so to speak.
3. Arduino Timers Control
You can configure & program the Arduino timer modules in two different ways. The first of which is bare-metal register access programming using the Timer control registers & the information provided in the datasheet. And this is exactly what we’ve discussed in the Arduino Timers & Timer Interrupts Tutorial.
The other method to control timer modules is to use Timer Libraries like the Arduino TimerOne Library. This is the topic of this tutorial, as we’ll discuss how to install and use TimerOne library. Which can be a lot easier to use than what we’ve done in the Arduino timer interrupts tutorial previously.
For more information about Arduino Timers, fundamental concepts, different timer operating modes, and code examples, it’s highly recommended to check out the tutorial linked below. It’s the ultimate guide for Arduino Timers.
This article will give more in-depth information about Arduino Timers, how timers work, different timer operating modes, practical use cases and code examples, and everything you’d ever need to know about Arduino Timers.
Arduino TimerOne Library
The Arduino TimerOne library is a community-contributed library that enables users to configure and use the 16-Bit Timer1 for generating & handling periodic interrupts and also to generate PWM signals with controllable frequency and duty cycle.
1- Using Arduino TimerOne Library
To use the Arduino TimerOne library, you need to include its header file in your code like this.
1 |
#include <TimerOne.h> |
Then you can use the provided APIs to configure the timer module, attach an interrupt handler to it, and so on. We’ll next explore the timing functions & PWM control functions in the Arduino TimerOne library.
2- Arduino TimerOne Time Functions
Timer1.initialize(period)
You must call this method first to use any of the other methods. You can optionally specify the timer’s period here (in microseconds), by default it is set at 1 second. Note that this disrupts the analogWrite() for digital pins 9 and 10 on Arduino UNO.
Here is an example of how to initialize Timer1 to have a period of 10ms.
1 |
Timer1.initialize(10000); // Fire An Interrupt Every 10ms |
Timer1.setPeriod(period)
Sets the period in microseconds. The minimum period or highest frequency this library supports is 1 microsecond or 1 MHz. The maximum period is 8388480 microseconds or about 8.3 seconds. Note that setting the period will change the attached interrupt and both pwm outputs’ frequencies and duty cycles simultaneously.
Here is an example of how to to set the period of Timer1 to 1ms.
1 |
Timer1.setPeriod(1000); |
Timer1.attachInterrupt(function, period)
Calls a function at the specified interval in microseconds. Note that you can optionally set the period with this function if you include a value in microseconds as the last parameter when you call it.
Here is an example of how to attachinterrupt to Timer1 periodic event so it executes the Timer1_ISR handler function at fixed periodicity of 1ms.
1 |
Timer1.attachInterrupt(Timer1_ISR, 1000); |
Timer1.detachInterrupt()
Disables the attached interrupt.
1 |
Timer1.detachInterrupt(); |
3- Arduino TimerOne PWM Functions
Timer1.pwm(pin, duty, period)
Generates a PWM waveform on the specified pin. Output pins for Timer1 are PORTB pins 1 and 2, so you have to choose between these two, anything else is ignored. On Arduino UNO, these are digital pins 9 and 10. Output pins for Timer3 are from PORTE and correspond to 2,3 & 5 on the Arduino Mega.
The duty cycle is specified as a 10 bit value, so anything between 0 and 1023. Note that you can optionally set the period with this function if you include a value in microseconds as the last parameter when you call it.
The TimerOne PWM outputs are only available for Arduino pins 9 & 10 only. Those are the hardware output pins (OC1A & OC1B) for Timer1 compare match outputs.
The duty cycle is only 10 bits despite the fact that the Timer1 module is a 16-bit timer and it can generate up to 16-Bits resolution PWM signal but resolution goes down as PWM frequency goes up. You can check the implementation here for your reference.
Here is an example of how to configure a PWM output channel on pin9 to generate a 50% duty cycle PWM signal at 1kHz frequency.
1 |
Timer1.pwm(9, 512, 1000); |
Timer1.setPwmDuty(pin, duty)
A fast shortcut for setting the pwm duty for a given pin if you have already set it up by calling the Timer1.pwm() function earlier. This avoids the overhead of (enabling pwm mode for the pin, setting the data direction register, checking for optional period adjustments, etc.) that are mandatory when you call the Timer1.pwm() function.
Here is an example for how to use it to change the duty cycle value to be 10% on output pin9.
1 |
Timer1.setPwmDuty(9, 102); |
Timer1.disablePwm(pin)
Turns PWM off for the specified pin so you can use that pin for something else. Here is how to use it to disable PWM output on pin9.
1 |
Timer1.disablePwm(9); |
4- Arduino TimerOne Other Useful Functions
Those are some other useful functions in the TimerOne library that you may occasionally use in different projects.
1 2 3 4 |
void stop(); // Pause Timer1 By Disabling The Clock void start(); // Resume Timer1 Clock void restart(); // Clear The Timer Ticks unsigned long read(); // Reads the time since last rollover (in μs) |
Arduino TimerOne Library Installation
You can download and install the Arduino TimerOne library manually from GitHub, or alternatively, install it within the Arduino IDE itself. Just open the library manager. Tools > Manage Libraries > Search for TimerOne. Then, click Install and let it finish the installation.
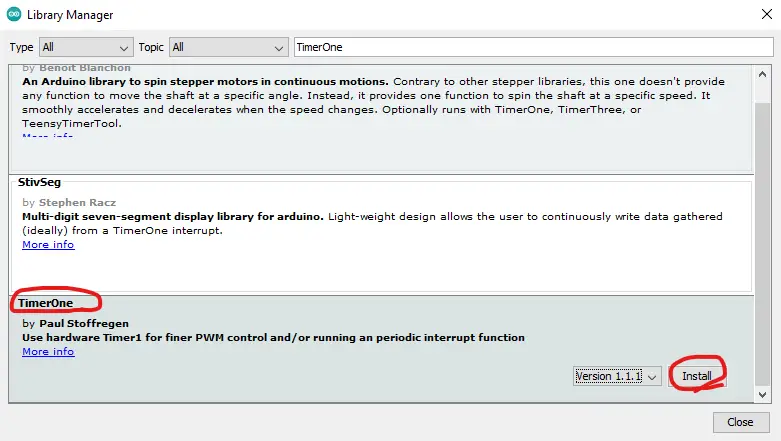
Now, you can easily use the Arduino TimerOne library and check the built-in examples for the library to help you get started.
We’ll move now to the practical code examples to test this Arduino TimerOne library for generating periodic timer interrupts.
Arduino TimerOne Timer Interrupt Example
In this example project, we’ll test the Arduino TimerOne library. We’ll create a 10ms periodic timer interrupt event, in which we’ll toggle an output LED pin. And we’ll measure the output timing using an oscilloscope to make sure that the timer library is working as expected.
Arduino TimerOne Timer Interrupt Example Code
Here is the full code listing for this Arduino TimerOne Example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
/* * LAB Name: Arduino TimerOne Library Example * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ #include <TimerOne.h> void Timer1_ISR(void) { digitalWrite(LED_BUILTIN, !digitalRead(LED_BUILTIN)); } void setup(void) { pinMode(LED_BUILTIN, OUTPUT); Timer1.initialize(10000); // Fire An Interrupt Every 10ms Timer1.attachInterrupt(Timer1_ISR); } void loop(void) { // Nothing To Do } |
Code Explanation
We should first include the TimerOne library.
1 |
#include <TimerOne.h> |
Timer1_ISR()
This is the timer interrupt handler function for the periodic 10ms interrupts. In which we’ll toggle the output LED pin.
1 2 3 4 |
void Timer1_ISR(void) { digitalWrite(LED_BUILTIN, !digitalRead(LED_BUILTIN)); } |
setup()
in the setup() function, we’ll set the LED pins’ mode to output and attach the Timer1_ISR function to the Timer1 set it to fire an interrupt every (10ms) time interval.
1 2 3 4 5 6 |
void setup(void) { pinMode(LED_BUILTIN, OUTPUT); Timer1.initialize(10000); // Fire An Interrupt Every 10ms Timer1.attachInterrupt(Timer1_ISR); } |
loop()
in the loop() function, nothing needs to be done.
Proteus Simulation
Here is the simulation result for this project on the Proteus (ISIS) simulator. The TinkerCAD simulation for this example will not produce a reliable behavior maybe due to inaccuracies in the microcontroller model in the simulation environment. The proteus simulation is way more accurate and behaves exactly like the real Arduino UNO board.
Moreover, we’ve got a better and much more powerful set of virtual measurement tools (oscilloscope, function generator, multimeters, virtual serial terminal, etc).
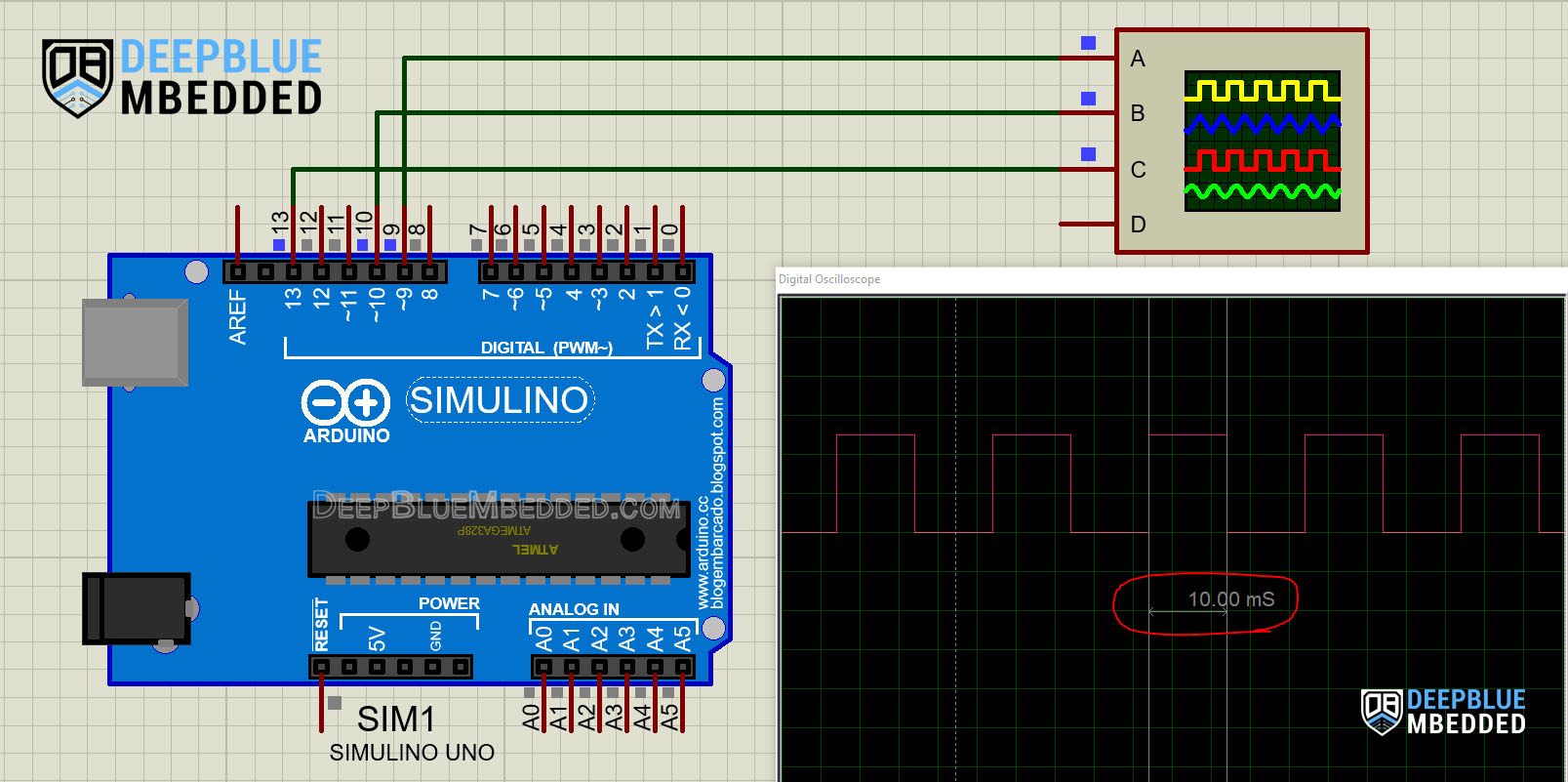
Check out the tutorial below to help you get started with simulating your Arduino projects in the Proteus simulation environment.
This article will provide you with more in-depth information about using proteus ISIS for Arduino projects simulation.
Testing Results
It shows a perfect 10ms pin toggle event exactly as we have expected and seen in the simulation.
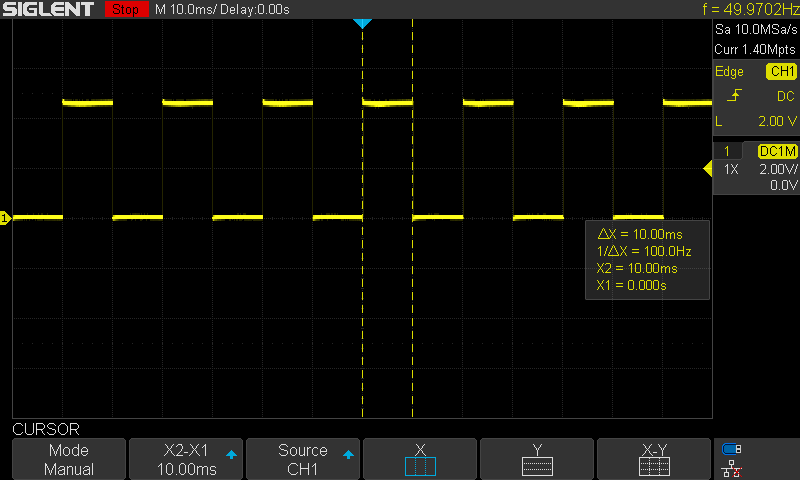
Arduino TimerOne 20kHz PWM Example
In this example project, we’ll test the Arduino TimerOne library for PWM output. There are so many applications in which we need the Arduino to generate 20kHz PWM output signal. Using Arduino TimerOne can be really helpful to achieve this requirement while maintaining a high PWM resolution.
We’ll control both PWM channels of TimerOne (pin9 and pin10) simultaneously. Each PWM output is 20kHz in frequency and the duty cycle will sweep from 0% to 100% (fade in) and from 100% down to 0% (fade out). The PWM output duty cycle on each channel will be the complement of the other (reverse effect).
Arduino TimerOne 20kHz PWM Output Example Code
Here is the full code listing for this Arduino TimerOne 20kHz PWM Output Example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
/* * LAB Name: Arduino TimerOne Library PWM Example * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ #include <TimerOne.h> void setup(void) { pinMode(9, OUTPUT); pinMode(10, OUTPUT); Timer1.initialize(50); Timer1.pwm(9, 0); Timer1.pwm(10, 1023); } void loop(void) { for (int i = 0; i < 1023; i++) { Timer1.setPwmDuty(9, i); Timer1.setPwmDuty(10, (1023 - i)); delay(1); } for (int i = 1023; i > 0; i--) { Timer1.setPwmDuty(9, i); Timer1.setPwmDuty(10, (1023 - i)); delay(1); } } |
Code Explanation
We should first include the TimerOne library.
1 |
#include <TimerOne.h> |
setup()
in the setup() function, we’ll set the PWM output pins’ mode (pin9 and 10). We’ll also set the PWM period to 50μs which means a PWM frequency of 20kHz. And we’ll finally set an initial value for PWM duty cycle on both channels.
1 2 3 4 5 6 7 8 |
void setup(void) { pinMode(9, OUTPUT); pinMode(10, OUTPUT); Timer1.initialize(50); Timer1.pwm(9, 0); Timer1.pwm(10, 1023); } |
loop()
in the loop() function, we’ll do the fade in and out effect on both channels. Each one will have the complementary value to the other (reverse effect).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
void loop(void) { for (int i = 0; i < 1023; i++) { Timer1.setPwmDuty(9, i); Timer1.setPwmDuty(10, (1023 - i)); delay(1); } for (int i = 1023; i > 0; i--) { Timer1.setPwmDuty(9, i); Timer1.setPwmDuty(10, (1023 - i)); delay(1); } } |
Proteus Simulation
Here is the simulation result for this project on the Proteus (ISIS) simulator. The TinkerCAD simulation for this example will not produce a reliable behavior maybe due to inaccuracies in the microcontroller model in the simulation environment. The proteus simulation is way more accurate and behaves exactly like the real Arduino UNO board.
Moreover, we’ve got a better and much more powerful set of virtual measurement tools (oscilloscope, function generator, multimeters, virtual serial terminal, etc).
Testing Results
Parts List
Here is the full components list for all parts that you’d need in order to perform the practical LABs mentioned here in this article and for the whole Arduino Programming series of tutorials found here on DeepBlueMbedded. Please, note that those are affiliate links and we’ll receive a small commission on your purchase at no additional cost to you, and it’d definitely support our work.
Download Attachments
You can download all attachment files for this Article/Tutorial (project files, schematics, code, etc..) using the link below. Please consider supporting my work through the various support options listed in the link down below. Every small donation helps to keep this website up and running and ultimately supports our community.
Arduino TimerOne Wrap Up
To conclude this tutorial, we’d like to highlight the fact that the Arduino TimerOne library is very easy to install and use. It provides users with useful APIs to configure and use the 16-Bit Timer1 for generating & handling periodic interrupts and also to generate PWM signals with controllable frequency and duty cycle.
This tutorial is a fundamental part of our Arduino Series of Tutorials because we’ll build on top of it to interface various sensors and modules with Arduino in other tutorials & projects. So make sure you get the hang of it and try all provided code examples & simulations (if you don’t have your Arduino Kit already).
If you’re just getting started with Arduino, you need to check out the Arduino Getting Started [Ultimate Guide] here.
This is the ultimate guide for getting started with Arduino for beginners. It’ll help you learn the Arduino fundamentals for Hardware & Software and understand the basics required to accelerate your learning journey with Arduino Programming.