In this tutorial, we’ll discuss the STM32 Internal Temperature Sensor Reading using the dedicated ADC channel that’s internally routed to the embedded temperature sensor. We’ll implement an example project for STM32 internal temperature sensor measurement and try to get accurate results as much as possible.
Near the end of this tutorial, we’ll also discuss some tips that will help you calibrate the SM32 internal temperature sensor and get the best results out of it. Without further ado, let’s get right into it!
Table of Contents
- STM32 Internal Temperature Sensor
- STM32 Internal Temperature Sensor Example Overview
- STM32 Internal Temperature Sensor Reading Example
- STM32 Internal Temperature Sensor Calibration
- Wrap Up
STM32 Internal Temperature Sensor
The STM32 microcontrollers have an internal temperature sensor that’s internally connected to a dedicated ADC input channel. It’s intended for use as an indicator of the MCU’s core temperature or as a backup to mitigate external ambient temperature sensor failure events. Therefore, it’s not very accurate for absolute temperature measurement as it’s not designed for that purpose. However, it can be very useful for detecting major temperature changes.
1. STM32 Internal Temperature Sensor Diagram
This is the block diagram for the STM32 internal temperature sensor that shows how it’s internally connected to a dedicated ADC channel as well as the internal analog reference voltage (VREFINT).
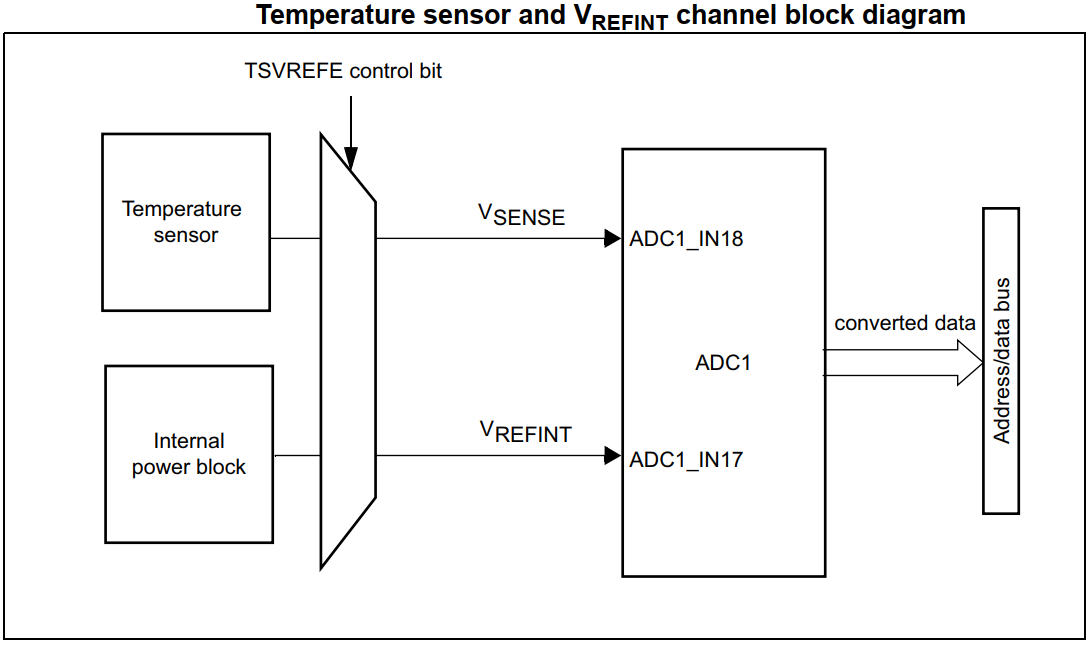
2. STM32 Internal Temperature Sensor Reading
To read the STM32’s internal temperature sensor, we’ll do the following steps:
- Enable The Temperature Sense ADC Channel
- Set its sampling time to 17µs or larger
- Start the ADC conversion
- Get the ADC reading and calculate the VSENSE voltage
- Obtain the temperature (in °C) using the equation shown below

Where the V25 is the voltage of the sensor’s output at (25°C) and it can be found in the “Electrical Specifications” in the MCU’s datasheet. The Average Slope is given in (mV/°C) and it’s also defined in the datasheet of the microcontroller.
For The STM32F103 target microcontroller, the Internal Temperature Sensor’s Parameters are as follows:
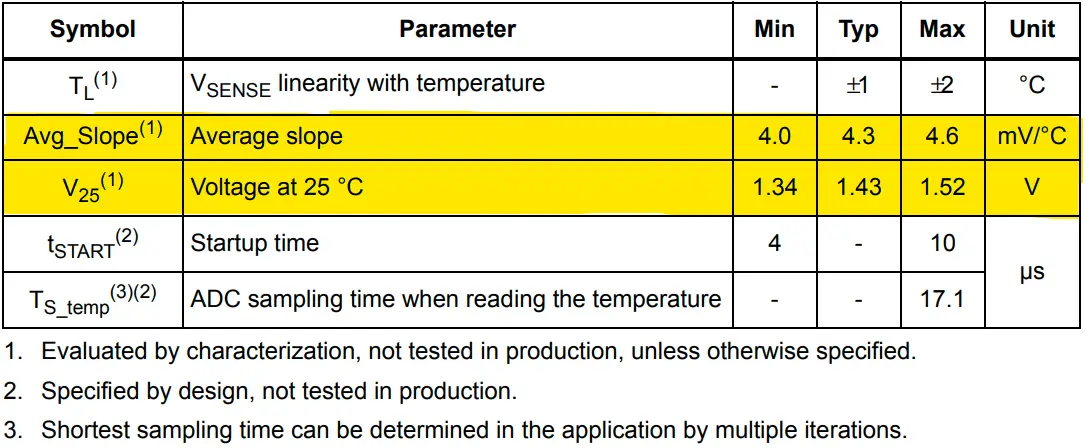
For The STM32F103 target microcontroller, the Internal Voltage Reference (VREFINT) Specifications are as follows:
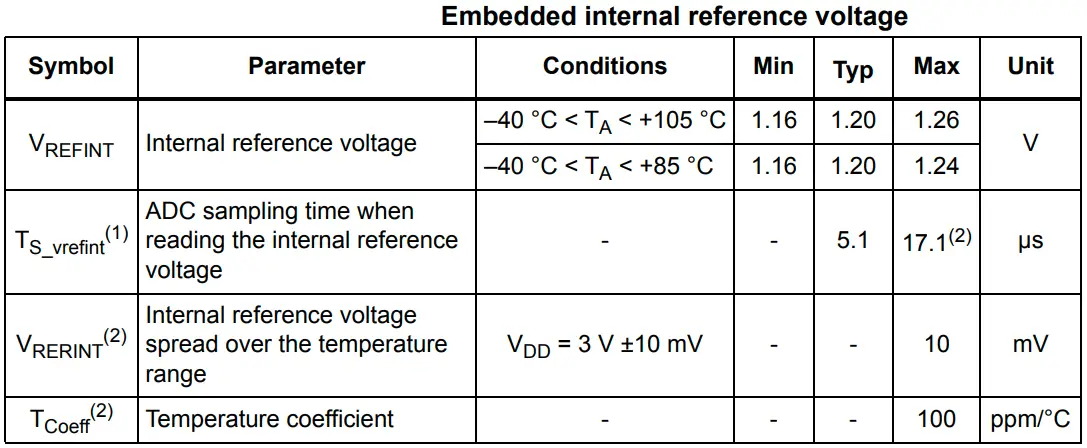
Please be advised, that you need to check the datasheet of your specific target STM32 microcontroller as it can have a different equation for calculating the temperature of the internal sensor. And of course, the parameters of the internal sensor & VREFINT may also vary from one target MCU to another.
Pay attention to the ADC’s clock rate and the sampling clock cycle count for the temperature sensor’s channel. It needs to have at least 17µs of sampling time to get reliable results.
STM32 Internal Temperature Sensor Example Overview
In this example project, we’ll create an STM32 ambient temperature measurement application using the internal temperature sensor. We’ll set the sampling rate for the temperature sensor to 50Hz (get 1 reading every 20ms) using a timer as a trigger source for the ADC channels. We’ll also continuously read the internal analog voltage reference value to correct for any variations in the supply voltage.
The calculated temperature values will be sent over UART to the PC’s serial monitor/plotter for visualization.
Example Project Steps Summary:
- Set up a new project, configure the RCC, clock tree, and enable SWD (serial wire debug)
- Enable the ADC’s temperature sensor channel in Regular Single-Conversion Mode With Timer3 TRGO event as a trigger source
- Enable the ADC’s internal reference voltage channel with similar settings to the VSENSE channel
- Set up Timer3 to generate an update event as a TRGO @ 20ms (50Hz rate) (so the ADC sampling rate will also be 50Hz)
- Set up a GPIO pin as an output pin, so we can toggle it in the ADC’s ISR to measure (verify) the ADC sampling rate
- Set up 1x UART channel to be used for sending serial data (text messages) to the PC every temperature measurement cycle
STM32 Internal Temperature Sensor Reading Example
Let’s now build this system step-by-step
Step #1
Open STM32CubeMX, create a new project, and select the target microcontroller.
Step #2
Configure The ADC1 Peripheral, Enable the regular channels:
Temperature Sense Channel and VREFINT Channel
Set the sampling time to 239.5 cycles (at 12MHz ADC clock, the 239.5 cycles = 20µs) which is the closest we can get to the specified 17µs sampling time
Select the Timer3 TRGO event as a trigger source. In the NVIC tab, enable the ADC1 global interrupt.
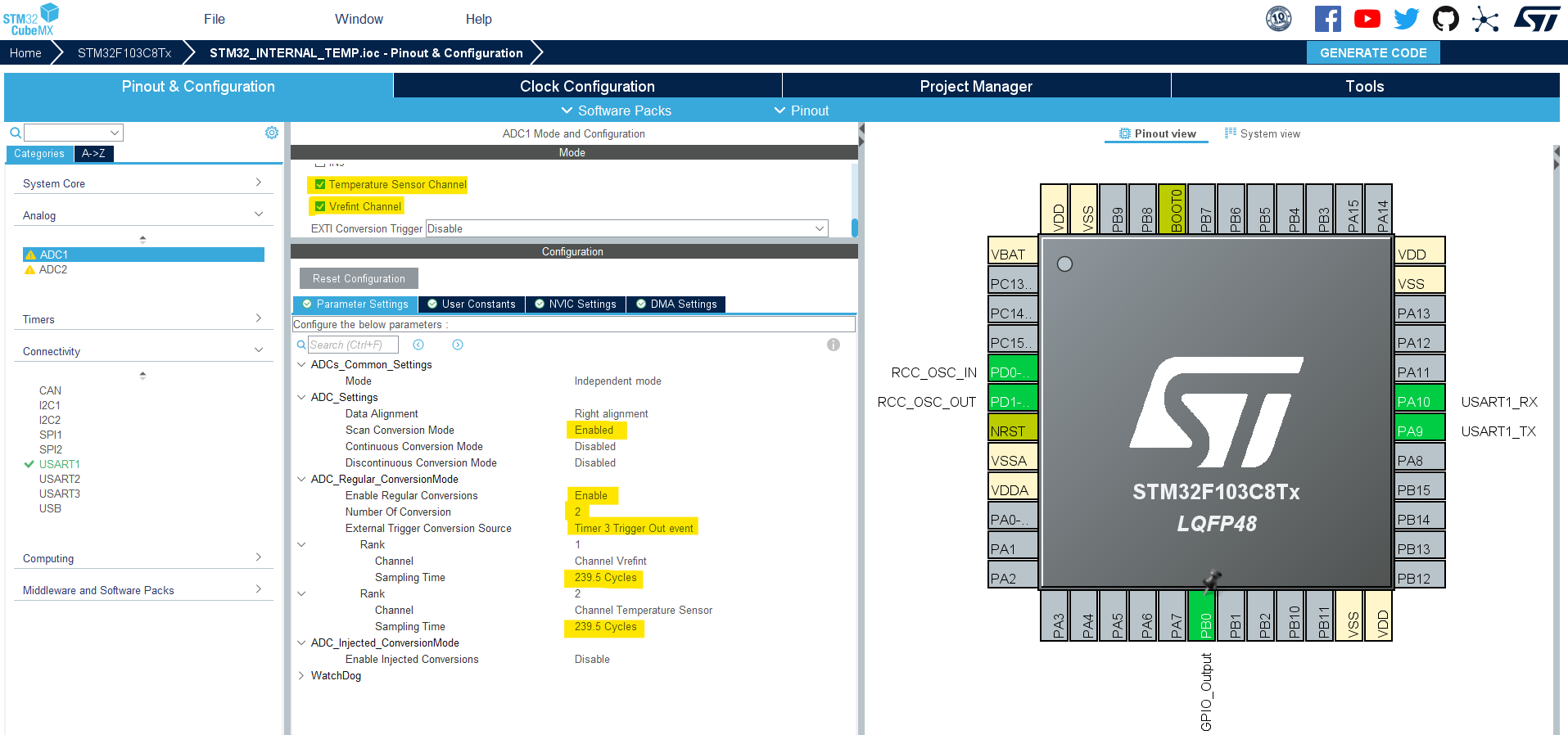
In the ADC’s DMA tab, add 1x DMA Channel for reading both channels automatically to memory locations in circular mode as shown below.
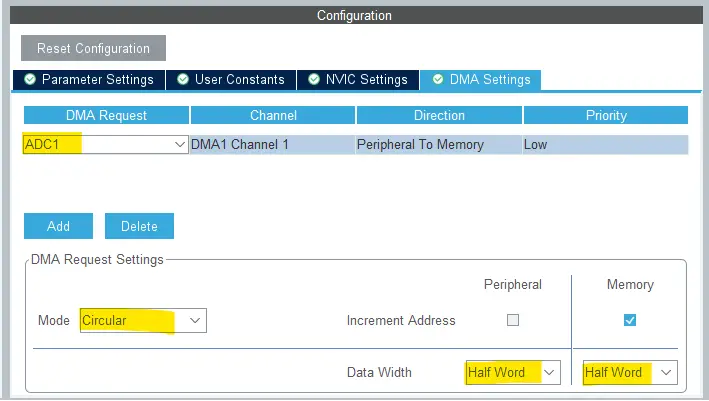
Step #3
For Timer3, we need to enable the internal clock for the timer (72MHz). Next, we need to set it in such a way that it generates an update event every 20ms to achieve the desired 50Hz ADC sampling rate. For this, you can use our STM32 Timer Calculator to get the required values for the Prescaler (PSC) and Auto-Reload Register (ARR) in order to achieve the 20ms update time interval. You’ll get PSC=23 & ARR=59999 from the calculator.
Finally, choose the TRGO event to be “Update Event“. And that’s all about it.
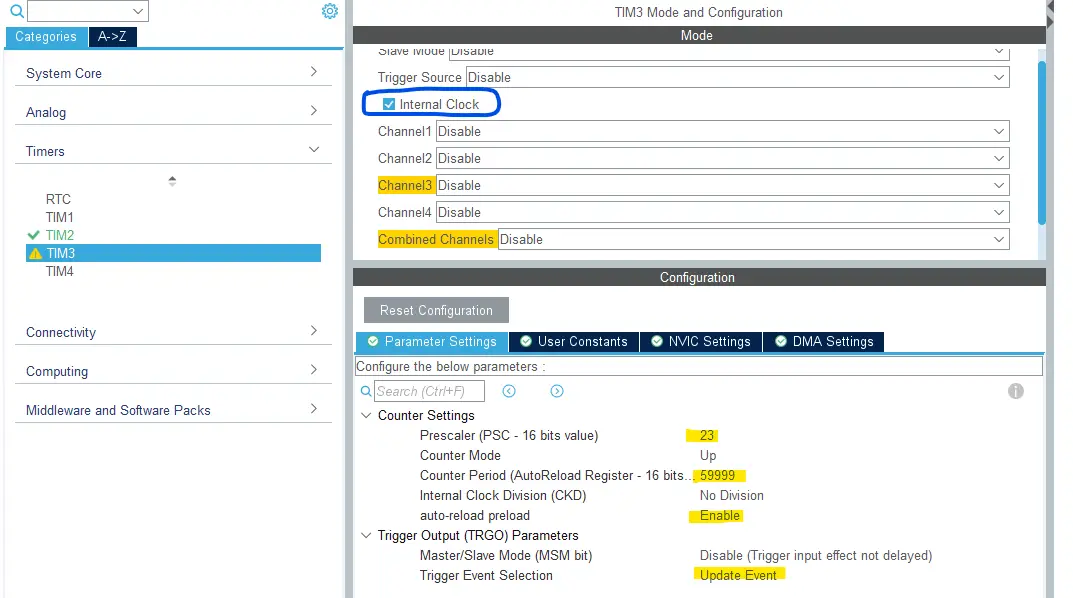
Step #4
Enable UART1 and leave the default configurations as is without a change (115200 baud rate).
Add 1x GPIO output pin for sampling rate verification (I’ve used the PB0 pin for this functionality).
Step #5
Go to the RCC clock configuration page and enable the HSE external crystal oscillator input.
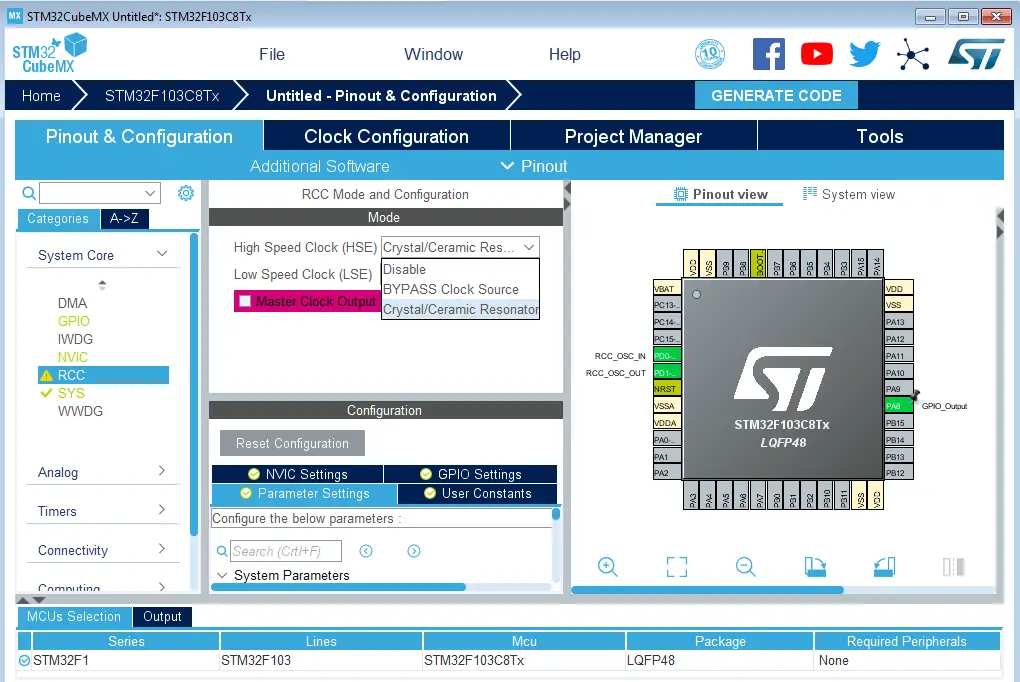
Step #6
Go to the clock configurations page, and select the HSE as a clock source, PLL output, and type in 72MHz for the desired output system frequency. Hit the “ Enter” key, and let the application solve for the required PLL dividers/multipliers to achieve the desired clock rate.
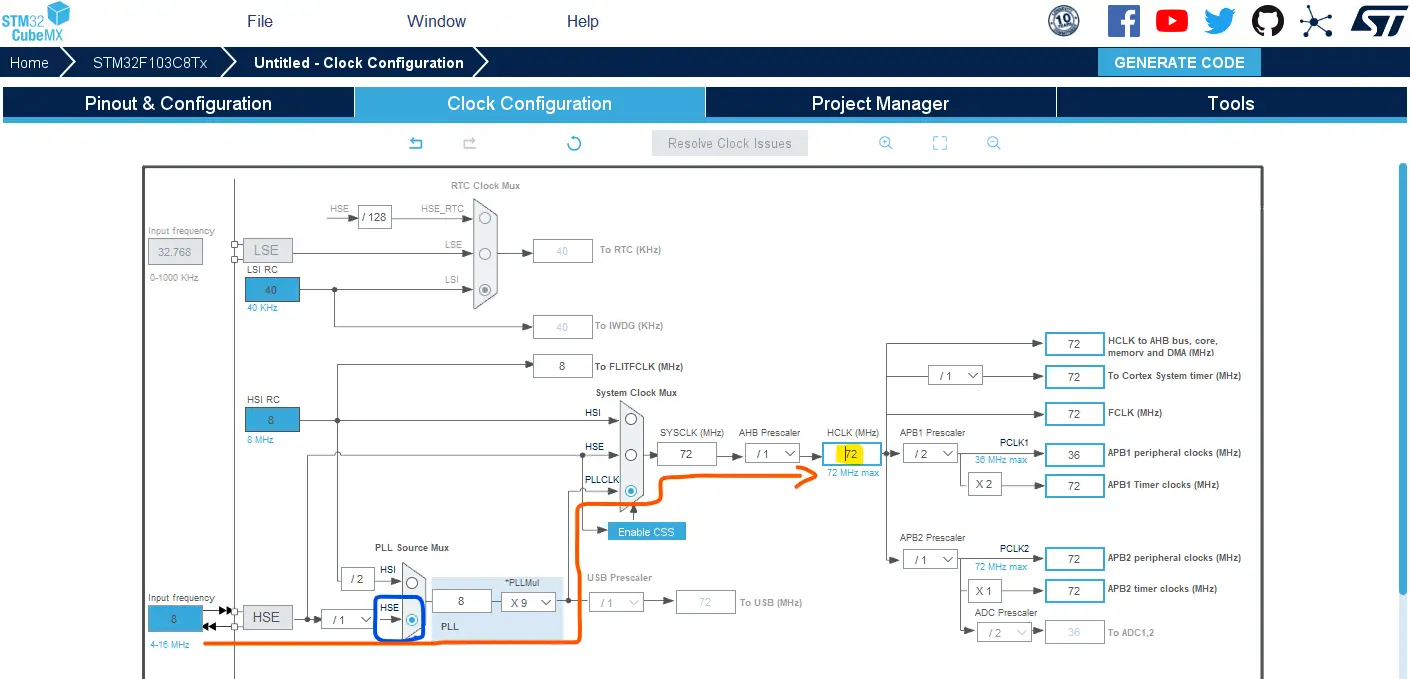
Step #7
Name & Generate The Project Initialization Code For CubeIDE or The IDE You’re Using.
STM32 Internal Temperature Sensor Example Code
Here is The Application Code For This LAB (main.c)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
/* * LAB Name: STM32 Internal Temperature Sensor Example * Author: Khaled Magdy * For More Info Visit: www.DeepBlueMbedded.com */ #include "main.h" #include <stdio.h> #include <string.h> #define AVG_SLOPE (4.3F) #define V_AT_25C (1.43F) #define V_REF_INT (1.2F) ADC_HandleTypeDef hadc1; DMA_HandleTypeDef hdma_adc1; TIM_HandleTypeDef htim3; UART_HandleTypeDef huart1; uint8_t UpdateEvent = 0; uint16_t AD_RES[2]; float Temperature, V_Sense, V_Ref; char TxBuffer[30]; void SystemClock_Config(void); static void MX_GPIO_Init(void); static void MX_DMA_Init(void); static void MX_ADC1_Init(void); static void MX_TIM3_Init(void); static void MX_USART1_UART_Init(void); int main(void) { HAL_Init(); SystemClock_Config(); MX_GPIO_Init(); MX_DMA_Init(); MX_ADC1_Init(); MX_TIM3_Init(); MX_USART1_UART_Init(); HAL_TIM_Base_Start(&htim3); // Start Timer3 (Trigger Source For ADC1) HAL_ADCEx_Calibration_Start(&hadc1); HAL_ADC_Start_DMA(&hadc1, (uint32_t*)AD_RES, 2); // Start ADC Conversion while (1) { if(UpdateEvent){ // Calculate The Temperature V_Ref = (float)((V_REF_INT * 4095.0)/AD_RES[0]); V_Sense = (float)(AD_RES[1] * V_Ref) / 4095.0; Temperature = (((V_AT_25C - V_Sense) * 1000.0) /AVG_SLOPE) + 25.0; // Send The Result To PC Over Serial Port sprintf(TxBuffer, "%f\r\n", Temperature); HAL_UART_Transmit(&huart1, (uint8_t*)TxBuffer, strlen(TxBuffer), 100); UpdateEvent = 0; } } } void HAL_ADC_ConvCpltCallback(ADC_HandleTypeDef* hadc) { HAL_GPIO_TogglePin(GPIOB, GPIO_PIN_0); // Toggle Interrupt Rate Indicator Pin UpdateEvent = 1; } |
Code Explanation
We need to start the Timer3 module by calling the HAL_TIM_Base_Start(&htim3) function so it can trigger the ADC every 20ms. And we also need to start the ADC in DMA mode at least once before the while(1) loop. Afterward, the ADC will be automatically triggered by the Timer3 module and we don’t need to call any function to start/trigger the ADC.
In the while(1) loop, we’ll calculate & update the temperature measurement every time the ADC completes the acquisition process. The result is therefore sent as a string over UART.
Finally, in the ADC interrupt handler (ISR), we’ll toggle the GPIO (B0) pin to measure/verify the ADC sampling rate (frequency).
STM32 Internal Temperature Sensor Example Testing
This test was conducted on my STM32F103 blue pill board with a heat gun as a source for heat that was applied on and off to see how fast the sensor reacts to ambient temperature change.
This is the PB0 pin as measured on my SDO showing that the ADC’s completion interrupt is fired exactly every 20ms (50Hz sampling rate).
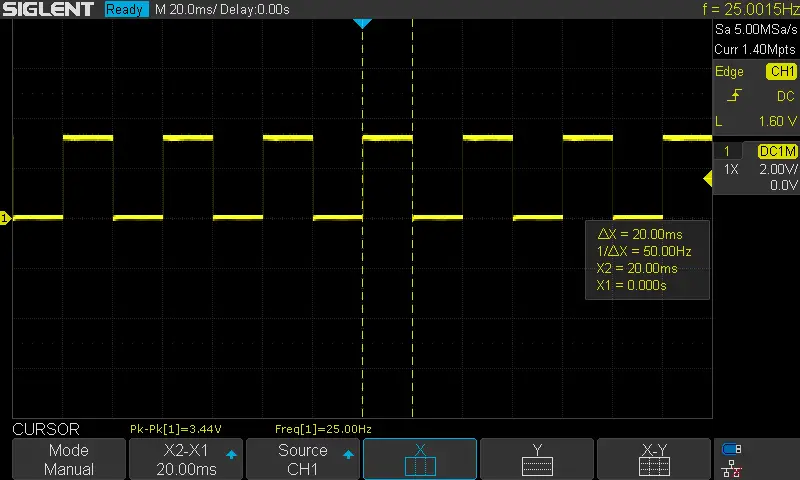
STM32 Internal Temperature Sensor Calibration
It’s very hard to get reliable results out of the STM32’s internal temperature sensor as it’s not designed to measure the ambient temperature but the internal core’s temperature. If your microcontroller is handling a computationally intensive workload, it’ll be a bit warmer than another one mostly IDLE most of the time.
However, to get the most out of the SM32 embedded internal temperature sensor we can do the following:
- Make sure you read the VREFINT every measurement cycle to accommodate for any change in the reference voltage
- Calibrate the ADC after each power-up
- Calibrate the temperature sensor using another reliable sensor (like the ds18B20) with an Arduino or something and place it as close as possible to the STM32 MCU chip and expose both of them to the same heating element. Adjust the (V25 & AvgSlope) parameters until you get a matching between the measurements of the embedded temperature sensor and the external dedicated (more accurate) sensor
Required Parts For STM32 Examples
All the example Code/LABs/Projects in this STM32 Series of Tutorials are done using the Dev boards & Electronic Parts Below:
QTY. | Component Name | Amazon.com | AliExpress | eBay |
1 | STM32-F103 BluePill Board (ARM Cortex-M3 @ 72MHz) | Amazon | AliExpress | eBay |
1 | Nucleo-L432KC (ARM Cortex-M4 @ 80MHz) | Amazon | AliExpress | eBay |
1 | ST-Link V2 Debugger | Amazon | AliExpress | eBay |
2 | BreadBoard | Amazon | AliExpress | eBay |
1 | LEDs Kit | Amazon & Amazon | AliExpress | eBay |
1 | Resistors Kit | Amazon & Amazon | AliExpress | eBay |
1 | Capacitors Kit | Amazon & Amazon | AliExpress & AliExpress | eBay & eBay |
1 | Jumper Wires Pack | Amazon & Amazon | AliExpress & AliExpress | eBay & eBay |
1 | Push Buttons | Amazon & Amazon | AliExpress | eBay |
1 | Potentiometers | Amazon | AliExpress | eBay |
1 | Micro USB Cable | Amazon | AliExpress | eBay |
★ Check The Links Below For The Full Course Kit List & LAB Test Equipment Required For Debugging ★
Download Attachments
You can download all attachment files for this Article/Tutorial (project files, schematics, code, etc..) using the link below. Please consider supporting our work through the various support options listed in the link down below. Every small donation helps to keep this website up and running and ultimately supports the whole community.
Wrap Up
In conclusion, we’ve explored how to set up the STM32 internal temperature sensor and use it to read the ambient temperature and make it as accurate as possible. You can build on top of the examples provided in this tutorial and/or explore the other temperature sensors that you can use with STM32 microcontrollers that you’ll find here on this SM32 Sensors Tutorials page.